Guide
The Complete Guide to Building LangChain Agents
Building applications with Large Language Models (LLMs) presents unique challenges, particularly in orchestrating complex tasks and managing memory. Developers often need frameworks to simplify these processes, allowing for more efficient and effective AI agent development. LangChain and LangGraph are popular frameworks that have gained attention for their capabilities in this area.
LangChain specializes in creating multi-step language processing workflows. It's a tool that helps in tasks like content generation and language translation by chaining together different language model operations.
On the other hand, LangGraph offers a flexible framework for building stateful applications. It handles complex scenarios involving multiple agents and facilitates human-agent collaboration with features like built-in statefulness, Human-in-the-loop Workflows and first-class streaming support.
Memory management is a crucial aspect when working with LLM-based agents. An AI agent's ability to retain and utilize information from previous interactions is essential for generating coherent and contextually appropriate responses. However, developers may encounter challenges such as limitations in context windows and maintaining consistent memory over prolonged interactions or tasks.
This article will explore the differences between LangChain and LangGraph, with a focus on how each framework addresses the challenges of memory management in AI agents. There will be practical examples of building agents using LangGraph. Additionally, we'll discuss recent advancements that have enhanced agent memory, offering insights into how these developments can impact your AI application.
Summary of key LangChain and LangGraph concepts
Overview of LangChain vs. LangGraph
When building applications with Large Language Models (LLMs), choosing the right framework can significantly impact your project's efficiency and scalability. While both LangChain and LangGraph aim to simplify the development of AI agents, they cater to different needs and complexities.
LangChain: simplifying LLM interactions
LangChain is an open-source framework designed to help developers create applications using LLMs. Its primary strength lies in building simple chains of language model interactions. Think of LangChain as a toolkit that allows you to link various language models and tasks together seamlessly. Whether you're working on a chatbot, content generator, or data processing workflow, LangChain provides the flexibility and modularity needed to compose multiple models and manage prompts effectively.
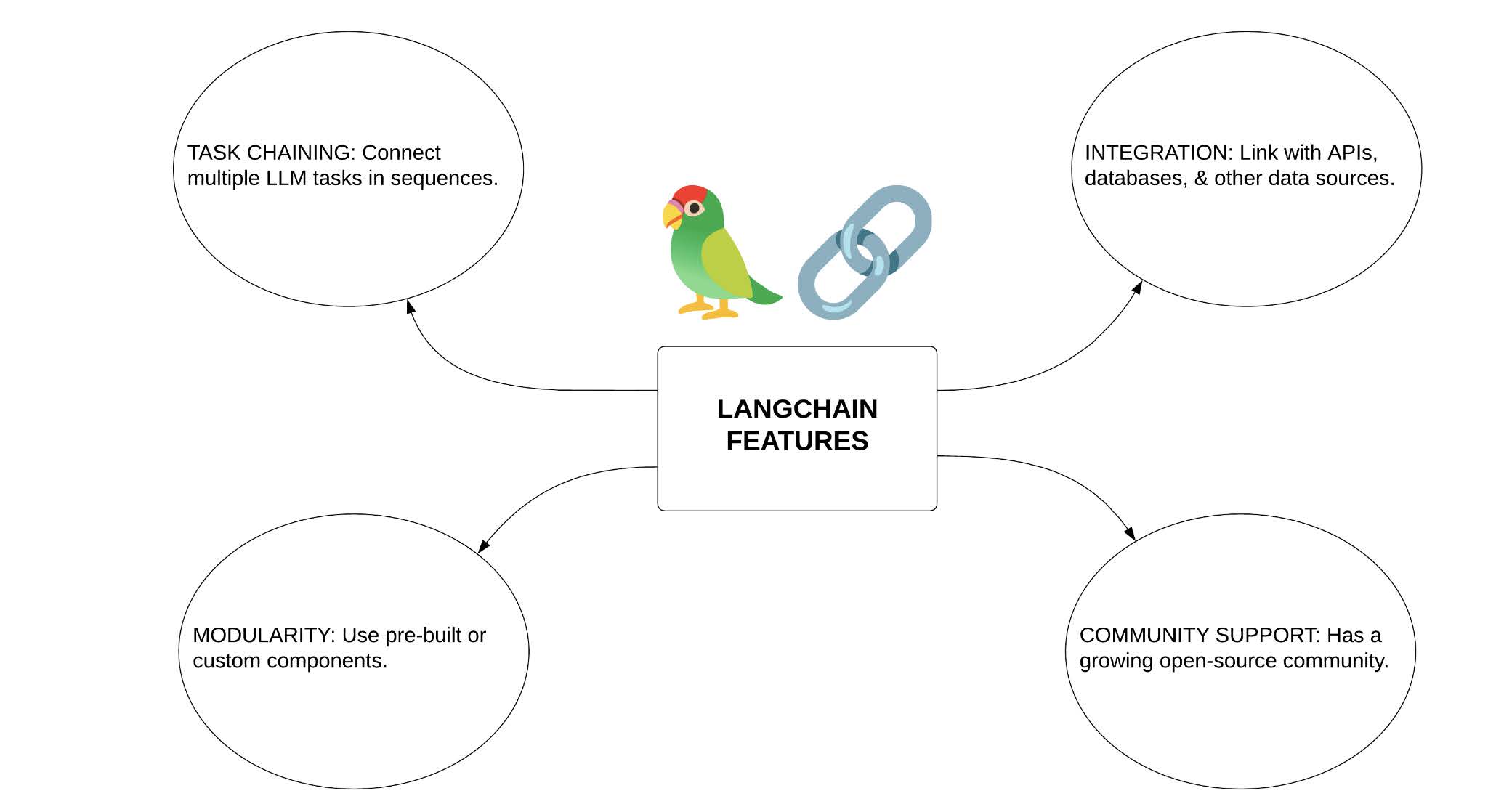
Key features of LangChain include:
- Task chaining: Easily connect multiple language model tasks in a sequence.
- Modularity: Use pre-built components or create custom ones to fit your specific needs.
- Integration: Connect with external data sources like APIs, databases, and files to enrich your applications.
- Community support: Being open-source, LangChain has a vibrant community that contributes modules and extensions, enhancing its capabilities.
LangGraph: orchestrating complex workflows
LangGraph is built to handle more sophisticated and intricate workflows. While LangChain excels in straightforward task chaining, LangGraph takes it a step further by offering a graph-based approach to orchestrate complex conversational flows and data pipelines. This makes LangGraph particularly suitable for projects that require managing multiple agents, conditional logic, and stateful interactions.
Key features of LangGraph include:
- Graph-based workflows: Visualize and manage task dependencies through nodes and edges, making it easier to handle complex interactions.
- Cyclical graphs: Support for cyclical workflows allows for dynamic decision-making and iterative processes within your applications.
- State management: Maintain persistent states across different nodes, enabling functionalities like pausing, resuming, and incorporating human-in-the-loop interactions.
- Integration with LangChain and LangSmith: LangGraph extends the capabilities of LangChain by seamlessly integrating with it, as well as with LangSmith for monitoring and optimization.
Key Concepts of LangGraph
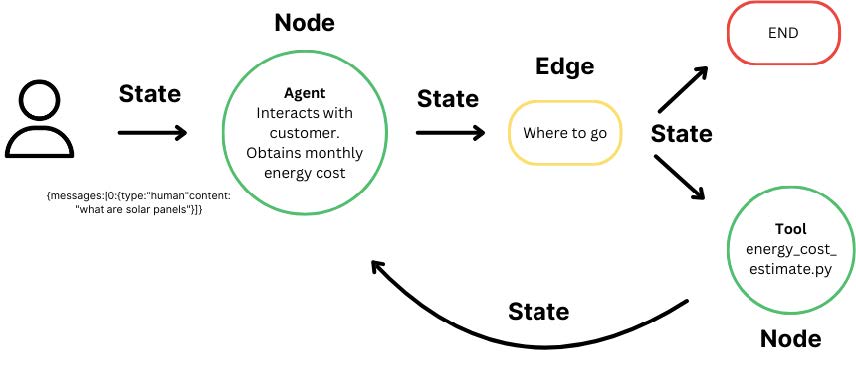
Understanding LangGraph's core concepts is essential to leveraging its full potential:
Cyclical graphs
Unlike linear workflows, cyclical graphs allow for loops and repeated interactions. This is crucial for managing tasks that require multiple iterations or conditional branching based on dynamic inputs.
Nodes and edges
The nodes represent the individual components of your workflow, such as LLMs, agents, or specific functions. Each node performs a distinct part of the overall task.
The edges define the connections between nodes, determining the flow of data and control. They can be conditional, directing the workflow based on certain criteria, or basic, following a straightforward path.
State management
LangGraph maintains a persistent state across different nodes, which means your application can pause and resume tasks without losing context. This is particularly useful for long-running processes or when human intervention is required at certain points.
Integration with LangChain and LangSmith
LangGraph doesn't work in isolation. It builds upon LangChain's capabilities, allowing you to incorporate complex workflows while still utilizing LangChain's modular task chaining. Additionally, integration with LangSmith provides tools for monitoring and optimizing your AI models, ensuring your applications run smoothly and efficiently.
Comparison of LangChain vs. LangGraph
LangChain has been around longer, earning a reputation for its versatility and strong community support. It's favored by developers who need a flexible framework to build a wide range of LLM applications without the overhead of managing complex workflows.
LangGraph, being newer, addresses the growing need to manage more sophisticated interactions and workflows. It attracts users who require a higher level of control and visibility over their processes, especially in scenarios where multiple agents and conditional logic are involved.
Migrating from LangChain Agents to LangGraph
As your projects grow in complexity, LangChain's straightforward task chaining becomes limiting. Transitioning to LangGraph can offer more control and flexibility for managing intricate workflows. Here's why and how you might consider making the switch.
Complex workflows
If your application involves multiple agents, conditional logic, or cyclical processes, LangGraph's graph-based approach can more effectively handle them.
State management and memory
For projects that require maintaining context across sessions or the ability to pause and resume tasks, LangGraph provides better state and memory management than LangChain. This make sures that AI agents can retain relevant information, for better continuity and responsiveness during complex user interactions.
Visualization and control
LangGraph’s visual workflow design makes it easier to understand and manage complex task dependencies, enhancing maintainability. LangGraph provides more granular control over agent actions and interactions.
Scalability issues
When LangChain starts to show limitations in handling large-scale or highly interactive workflows.
Integration requirements
When integrating with other tools like LangSmith for monitoring and optimization becomes essential.
Migration example - converting a LangChain agent to LangGraph
Let's walk through a simple example of migrating a LangChain-based chatbot to a LangGraph-based implementation. This will illustrate the practical steps and highlight the benefits of using LangGraph.
LangChain chatbot
from langchain import LLMChain
from langchain.llms import OpenAI
# Initialize the language model
llm = OpenAI(api_key='your-api-key')
# Define a simple chain
chain = LLMChain(llm=llm, prompt="Hello, how can I assist you today?")
# Run the chain
response = chain.run()
print(response)
LangGraph chatbot
from langgraph.graph import StateGraph
from langgraph.graph.message import add_messages
from langchain.llms import OpenAI
from typing import Annotated
from typing_extensions import TypedDict
from langchain_core.messages import HumanMessage
class State(TypedDict):
messages: Annotated[list, add_messages]
# Initialize the state graph
graph_builder = StateGraph(State)
# Initialize the language model
llm = OpenAI(api_key="you-api-key") # Replace with your actual API key
# Define the chatbot function
def chatbot(state: State):
# Extract the content of the last message
user_message = state["messages"][-1].content
response = llm.invoke(user_message)
return {"messages": [HumanMessage(content=response)]}
# Add the chatbot node
graph_builder.add_node("chatbot", chatbot)
# Set entry and finish points
graph_builder.set_entry_point("chatbot")
graph_builder.set_finish_point("chatbot")
# Compile the graph
graph = graph_builder.compile()
# Interactive loop
while True:
user_input = input("User: ")
if user_input.lower() in ["quit", "exit", "q"]:
print("Goodbye!")
break
for event in graph.stream({"messages": ("user", user_input)}):
for value in event.values():
print("Assistant:", value["messages"][-1][1])

Explanations:
- State definition: in LangGraph, we define a State that keeps track of the conversation messages. This persistent state allows the chatbot to remember previous interactions.
- Graph initialization: we create a StateGraph and add a node named "chatbot" which handles the interaction logic using the language model.
- Node function: the chatbot function takes the current state, processes the latest user message, and generates a response using the LLM.
- Setting entry and finish points: we designate the "chatbot" node as both the entry and finish point, meaning all interactions start and end with this node.
- Interactive loop: the loop allows for continuous user interaction, streaming responses from the graph and maintaining the conversation state.
While LangGraph requires more lines of code for simple tasks, this added complexity enables it to handle more advanced workflows. Its graph-based design allows for branching, looping, and conditional logic, making it ideal for complex, real-world scenarios.
LangGraph features
LangGraph offers a set of features that make it easier to build and manage complex workflows with LLMs. In this section, we'll see into some of its key capabilities, including cycles, branching, persistent state management, and human-in-the-loop workflows.
Cycles and branching
LangGraph allows you to implement loops and conditional logic within your workflows, enabling agents to handle more dynamic and complex tasks. By representing each agent or function as a node and defining the flow between them with edges, you can create workflows that branch based on specific conditions or repeat certain steps as needed.
For example, consider a workflow where an agent must process user input, perform a series of checks, and decide whether to continue processing or end the task based on the input length. The code below demonstrates the same.
from langgraph.graph import StateGraph, MessagesState, START, END
# Define the state structure
class State(TypedDict):
messages: Annotated[list, add_messages]
# Define node functions
def node1(state: State):
input_msg = state['messages'][-1]['content']
response = f"Received: {input_msg}"
return {"messages": [response]}
def node2(state: State):
input_msg = state['messages'][-1]['content']
if len(input_msg) > 50:
return {"next_node": "tool"}
return {"next_node": "__end__"}
def tool(state: State):
input_msg = state['messages'][-1]['content']
processed = input_msg.upper()
return {"messages": [processed]}
# Initialize the StateGraph
graph_builder = StateGraph(MessagesState)
# Add nodes to the graph
graph_builder.add_node("Node-1", node1)
graph_builder.add_node("Node-2", node2)
graph_builder.add_node("tool", tool)
# Define edges
graph_builder.add_edge(START, "Node-1")
graph_builder.add_edge("Node-1", "Node-2")
graph_builder.add_conditional_edges(
source="Node-2",
path=lambda state: "tool" if len(state['messages'][-1]['content']) > 50 else "__end__",
path_map={
"tool": "tool",
"__end__": "__end__"
}
)
graph_builder.add_edge("tool", END)
# Compile the graph
graph = graph_builder.compile()
# Run the graph with sample input
inputs = {"messages": [{"role": "human", "content": "This is a sample input that is sufficiently long to trigger the tool node."}]}
for output in graph.stream(inputs):
for key, value in output.items():
print(f"'{key}':\n---\n{value}\n~~~~~~~~\n")
Persistent state management
Managing the state across different nodes is crucial for maintaining context and ensuring the workflow can resume seamlessly after interruptions. LangGraph handles this through its persistent state management, which allows you to save and restore the state at any point in the workflow. Here's how you can implement persistent state management:
from langgraph.graph import StateGraph, MessagesState
from langgraph.checkpoint.sqlite import SqliteSaver
# Initialize the checkpointer
memory = SqliteSaver.from_conn_string("sqlite:///workflow_state.db")
# Compile the graph with persistence
graph = graph_builder.compile(checkpointer=memory)
# To resume from a saved state
thread_config = {"configurable": {"thread_id": "1"}}
for event in graph.stream(inputs, thread_config, stream_mode="values"):
for key, value in event.items():
print(f"'{key}':\n---\n{value}\n~~~~~~~~\n")
# The state is automatically saved after each step, allowing you to pause and resume as needed.
Let's take another example: follow-up question handling
A chatbot needs to handle a conversation where users can ask follow-up questions, and the bot references past messages stored in a persistent state.
from langgraph.graph import StateGraph, MessagesState, START, END
from langgraph.checkpoint.sqlite import SqliteSaver
from langchain_core.messages import HumanMessage
from typing_extensions import TypedDict
# Define the state structure
class State(TypedDict):
messages: list
# Initialize the persistent memory saver
memory_saver = SqliteSaver.from_conn_string("sqlite:///conversation_history.db")
# Define the chatbot node function
def chatbot_with_context(state: State):
# Retrieve conversation history from state
history = "\n".join([msg["content"] for msg in state["messages"]])
# Get the latest user input
user_message = state["messages"][-1]["content"]
# Generate a response using history
response = f"I remember you said: {history}. Now, you're asking: {user_message}"
return {"messages": [{"role": "assistant", "content": response}]}
# Build the graph
graph_builder = StateGraph(MessagesState)
graph_builder.add_node("ChatbotWithContext", chatbot_with_context)
graph_builder.add_edge(START, "ChatbotWithContext")
graph_builder.add_edge("ChatbotWithContext", END)
graph = graph_builder.compile(checkpointer=memory_saver)
# Simulate a conversation with follow-up questions
inputs = {
"messages": [
{"role": "user", "content": "What are your capabilities?"},
{"role": "user", "content": "Can you explain how memory works?"}
]
}
for event in graph.stream(inputs, stream_mode="values"):
for key, value in event.items():
print(f"{key}: {value['messages'][-1]['content']}")
Below is an explanation of the code:
- Persistent State Management:
- SqliteSaver ensures the state (conversation history) is saved after every interaction.
- If the graph is interrupted, the state can be restored seamlessly.
- Conversation History:
- The chatbot retrieves past user messages from the state["messages"] object and incorporates them into the response.
- Follow-Up Handling:
- Each user message is stored, enabling the bot to refer to previous interactions for generating context-aware replies.
Human-in-the-loop workflows
Incorporating human intervention into automated workflows can enhance the quality and accuracy of the outcomes. LangGraph supports human-in-the-loop (HIT) functionality, allowing humans to approve or modify actions planned by the agent before they are executed.
Collecting feedback
You can integrate human nodes within LangGraph to gather feedback and refine workflow outcomes. This ensures that critical decisions are reviewed by a human, adding an extra layer of reliability.
Editor node implementation
Using LangGraph, you can create nodes that involve humans in the decision-making process. For instance, an editor node can refine responses based on human feedback, improving the overall user experience.
Agentic human interaction
LangGraph also supports more dynamic HIT systems, where tools like HumanInputRun enable multiple interactions and refinements throughout the workflow. This is particularly useful for complex tasks that require iterative improvements.
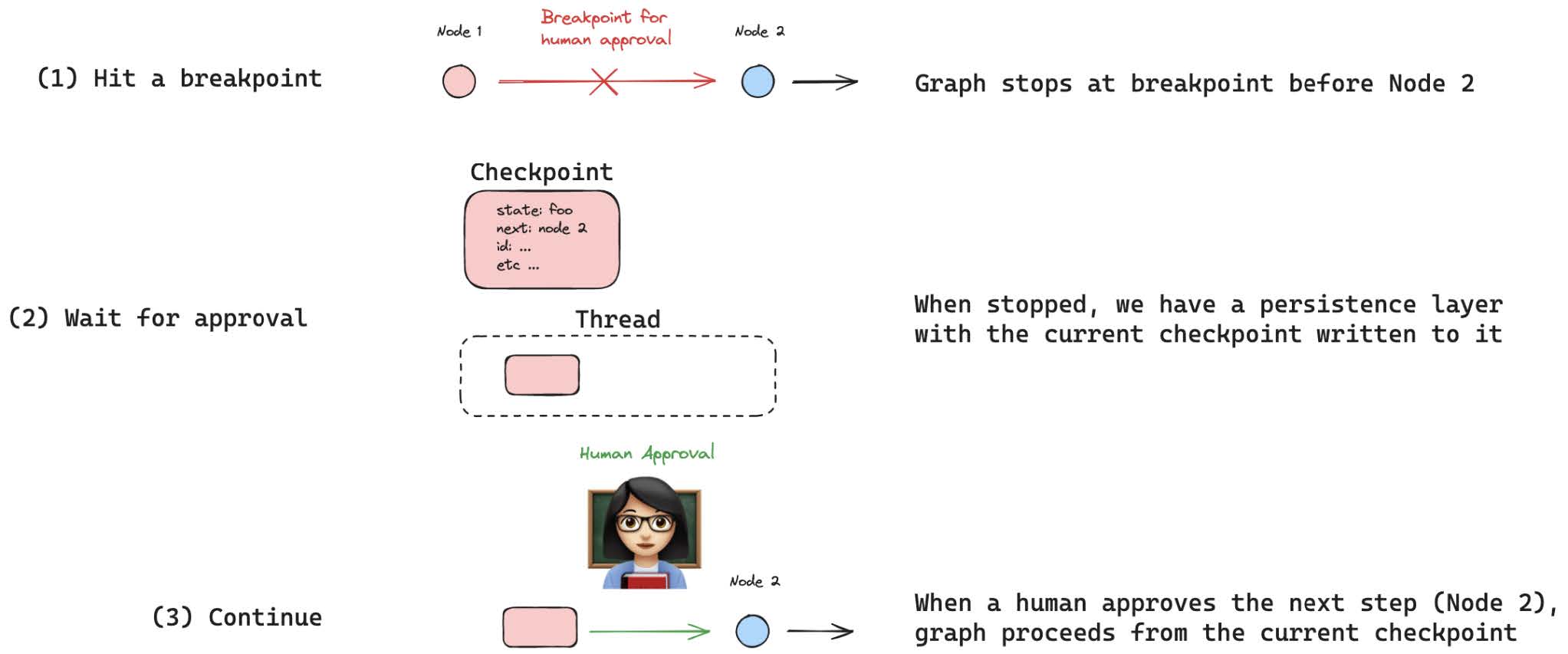
Code tutorial: adding human-in-the-loop nodes
Here's an example of how to add human-in-the-loop functionality to your workflow:
from langgraph.graph import StateGraph, MessagesState, START, END
from langgraph.graph.message import HumanMessage, SystemMessage
# Define the state structure
class State(TypedDict):
messages: Annotated[list, add_messages]
# Define node functions
def node1(state: State):
user_input = state['messages'][-1]['content']
response = f"Processing: {user_input}"
return {"messages": [response]}
def human_review(state: State):
# Simulate human approval
approval = input("Do you approve the action? (yes/no): ")
if approval.lower() == "yes":
return {"next_node": "node2"}
return {"next_node": "__end__"}
def node2(state: State):
return {"messages": ["Action approved and executed."]}
# Initialize the StateGraph
graph_builder = StateGraph(MessagesState)
# Add nodes to the graph
graph_builder.add_node("Node-1", node1)
graph_builder.add_node("Human-Review", human_review)
graph_builder.add_node("Node-2", node2)
# Define edges
graph_builder.add_edge(START, "Node-1")
graph_builder.add_edge("Node-1", "Human-Review")
graph_builder.add_conditional_edges(
source="Human-Review",
path=lambda state: "node2" if "approve" in state['messages'][-1]['content'].lower() else "__end__",
path_map={
"node2": "Node-2",
"__end__": "__end__"
}
)
graph_builder.add_edge("Node-2", END)
# Compile the graph
graph = graph_builder.compile()
# Run the graph with sample input
inputs = {"messages": [{"role": "human", "content": "Please execute the task."}]}
for output in graph.stream(inputs):
for key, value in output.items():
print(f"'{key}':\n---\n{value}\n~~~~~~~~\n")
In this example:
- Node-1 processes the initial user input.
- Human-Review pauses the workflow to ask for human approval.
- Depending on the response, the workflow either proceeds to Node-2 or ends.
This setup ensures that critical actions are vetted by a human, enhancing the reliability of the workflow.
Building single and multi-agent workflows
Building a single-agent workflow in LangGraph is straightforward and demonstrates the core concepts of the framework, such as state management and graph-based workflows. By using a graph-based design, LangGraph structures tasks as nodes and transitions as edges, providing a clear and flexible workflow architecture. Additionally, it highlights the real-time execution flow, where state updates seamlessly propagate through the graph.
Here's a step-by-step guide to building a basic chatbot.
Define the State
The state structure holds the conversation messages, maintaining context throughout the interaction.
from typing import Annotated, TypedDict
from langgraph.graph import StateGraph, MessagesState, START, END
from langgraph.graph.message import add_messages
class State(TypedDict):
messages: Annotated[list, add_messages]
Create Node Functions
Define how the agent processes incoming messages. In this example, the agent simply echoes the user's input.
def receive_message(state: State):
user_input = state['messages'][-1]['content']
response = f"Echo: {user_input}"
return {"messages": [response]}
Build the Graph
Construct the workflow by adding nodes and defining the flow from start to end.
graph_builder = StateGraph(MessagesState)
graph_builder.add_node("Receive", receive_message)
graph_builder.add_edge(START, "Receive")
graph_builder.add_edge("Receive", END)
graph = graph_builder.compile()
Run the Chatbot
Initiate the chatbot with a user message and stream the responses.
from langchain_core.messages import HumanMessage
inputs = {"messages": [HumanMessage(content="Hello!")]}
for output in graph.stream(inputs):
for key, value in output.items():
print(f"'{key}': {value['messages'][-1]}")
Multi-Agent Systems
For more complex applications, LangGraph supports multi-agent workflows where different agents handle specific tasks. Here's how to build a multi-agent system with a router agent directing queries to the appropriate expert agents.
Define the State
Similar to the single-agent workflow, the state holds conversation messages.
class State(TypedDict):
messages: Annotated[list, add_messages]
Create Agent Functions
Define agents for routing, weather, and news. The router directs queries to the appropriate agent based on the user's input.
def router_agent(state: State):
user_input = state['messages'][-1]['content']
if "weather" in user_input.lower():
return {"next_node": "WeatherAgent"}
elif "news" in user_input.lower():
return {"next_node": "NewsAgent"}
return {"next_node": "__end__"}
def weather_agent(state: State):
return {"messages": ["The weather is sunny today!"]}
def news_agent(state: State):
return {"messages": ["Here are the latest news headlines..."]}
Build the Graph
Set up the workflow by adding nodes and defining conditional paths based on the router's decisions.
graph_builder = StateGraph(MessagesState)
graph_builder.add_node("Router", router_agent)
graph_builder.add_node("WeatherAgent", weather_agent)
graph_builder.add_node("NewsAgent", news_agent)
graph_builder.add_edge(START, "Router")
graph_builder.add_conditional_edges(
source="Router",
path=lambda state: "WeatherAgent" if "weather" in state['messages'][-1]['content'].lower() else ("NewsAgent" if "news" in state['messages'][-1]['content'].lower() else "__end__"),
path_map={
"WeatherAgent": "WeatherAgent",
"NewsAgent": "NewsAgent",
"__end__": "__end__"
}
)
graph_builder.add_edge("WeatherAgent", END)
graph_builder.add_edge("NewsAgent", END)
graph = graph_builder.compile()
Run the multi-agent workflow
Provide user input and observe how the router directs the query to the appropriate agent.
inputs = {"messages": [HumanMessage(content="Tell me the weather today.")]}
for output in graph.stream(inputs):
for key, value in output.items():
print(f"'{key}': {value['messages'][-1]}")
Output:
'Router': {"next_node": "WeatherAgent"}
~~~~~~~~
'WeatherAgent': The weather is sunny today!
~~~~~~~~
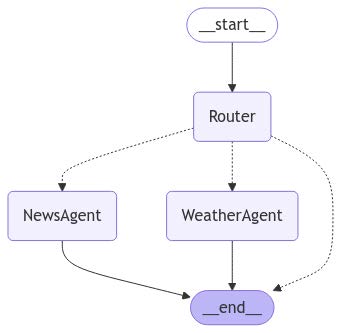
This setup allows the router agent to direct user queries to the appropriate expert agent based on the input, enabling more specialized and accurate responses.
Persistence and state management
In any AI workflow, managing and retaining context is essential for seamless interactions. LangGraph addresses this need with robust persistence and state management capabilities, ensuring workflows can maintain context and recover gracefully from interruptions.
Short-term memory
LangGraph efficiently manages short-term memory within conversations using state checkpoints. This approach is ideal for scenarios like basic customer support, where maintaining the context of the current interaction is sufficient.
Example - basic customer support
from langgraph.graph import StateGraph, MessagesState, START, END
from langchain_core.messages import HumanMessage
class State(TypedDict):
messages: Annotated[list, add_messages]
def support_agent(state: State):
user_query = state['messages'][-1]['content']
response = f"Support: How can I help you with '{user_query}'?"
return {"messages": [response]}
graph_builder = StateGraph(MessagesState)
graph_builder.add_node("SupportAgent", support_agent)
graph_builder.add_edge(START, "SupportAgent")
graph_builder.add_edge("SupportAgent", END)
graph = graph_builder.compile()
inputs = {"messages": [HumanMessage(content="I need help with my account.")]}
for output in graph.stream(inputs):
for key, value in output.items():
print(f"'{key}': {value['messages'][-1]}")
Limitations of short-term memory
As conversations grow, so does the chat history. This can lead to performance issues due to the increasing size of the context window. Managing this growth is essential to maintain efficiency.
Growing chat history
Long conversations result in a large accumulation of messages, which can slow down processing and increase costs. To address this, developers need strategies to manage and optimize chat history.
Need for pruning and selecting relevant history
Implementing pruning techniques, such as summarizing past messages or discarding less relevant ones, helps keep the chat history manageable. Maintaining essential context while reducing the overall state size ensures both performance and relevance.
Long-term memory in LangGraph
Recent updates in LangGraph introduce capabilities for long-term memory across multiple threads. This allows AI agents to retain information over extended periods, enhancing their ability to provide consistent and context-aware responses.
Imagine building a personalized customer support chatbot. The chatbot needs to remember user preferences and past interactions across sessions, such as previously reported issues, preferred communication styles, or product preferences.
Challenges of long-term memory
Implementing long-term memory in AI agents presents several challenges:
- Relevance Maintenance: Over time, the volume of stored information can become overwhelming, making it difficult to ensure that only pertinent data is retained. Without effective management, the agent might struggle to differentiate between essential and irrelevant information.
- Data Freshness: Information can become outdated or less relevant as contexts change. Keeping the memory updated requires mechanisms to periodically review and refresh stored data.
- Resource Optimization: Storing extensive histories can lead to increased resource consumption, affecting both performance and cost. Efficient memory management strategies are necessary to balance the depth of memory with resource usage.
{{banner-large-1="/banners"}}
How Zep addresses long-term memory challenges
Zep integrates seamlessly with LangGraph to tackle these challenges, giving a robust solution for managing long-term memory in AI applications. Here's how it works:
- Persistent storage: It comes with persistent storage solutions that allow AI agents to save and retrieve information across different sessions. This persistence ensures that the agent can maintain context even after interruptions or restarts.
- Efficient retrieval: With Zep, retrieving relevant facts becomes very efficient. The system can quickly access pertinent information without processing the entire history, thereby optimizing performance.
- Privacy and security: It emphasizes data privacy, ensuring that user information is handled securely. This focus is crucial for applications that deal with sensitive or personal data.
- Framework: Zep's framework-agnostic approach means it can integrate with various AI frameworks, including LangGraph, without requiring significant changes to existing workflows.
Code example of Zep integration with LangGraph
Integrating Zep with LangGraph enables persistent user memory, allowing agents to recall information across sessions. Below is an example that demonstrates this integration:
from langgraph.graph import StateGraph, MessagesState, START, END
from langgraph.checkpoint.memory import MemorySaver
from langgraph.prebuilt import ToolNode
from langchain_core.messages import HumanMessage
from zep_cloud.client import AsyncZep
from zep_cloud import Message
import asyncio
# Initialize Zep
zep = AsyncZep(api_key=os.environ.get('ZEP_API_KEY'))
# Define the state structure
class State(TypedDict):
messages: Annotated[list, add_messages]
user_name: str
session_id: str
# Define a tool for searching facts
@tool
async def search_facts(state: State, query: str, limit: int = 5):
return await zep.memory.search_sessions(
user_id=state['user_name'],
text=query,
limit=limit,
search_scope="facts"
)
tools = [search_facts]
tool_node = ToolNode(tools)
# Define the chatbot function
async def chatbot(state: State):
facts = await zep.memory.get(state["session_id"])
facts_string = "\n".join([f.fact for f in facts.facts]) if facts.relevant_facts else "No facts available."
response = f"Based on your history:\n{facts_string}"
return {"messages": [response]}
# Build the graph
graph_builder = StateGraph(State)
graph_builder.add_node("Chatbot", chatbot)
graph_builder.add_node("SearchFacts", tool_node)
graph_builder.add_edge(START, "Chatbot")
graph_builder.add_conditional_edges(
source="Chatbot",
path=lambda state: "SearchFacts" if "search" in state['messages'][-1]['content'].lower() else "__end__",
path_map={
"SearchFacts": "SearchFacts",
"__end__": "__end__"
}
)
graph_builder.add_edge("SearchFacts", END)
graph = graph_builder.compile()
# Run the graph with Zep integration
async def run_with_zep():
user_name = 'User_' + uuid.uuid4().hex[:4]
session_id = uuid.uuid4().hex
await zep.user.add(user_id=user_name)
await zep.memory.add_session(session_id=session_id, user_id=user_name)
inputs = {
"messages": [HumanMessage(content="Hello, I need some information.")],
"user_name": user_name,
"session_id": session_id
}
async for output in graph.stream(inputs):
for key, value in output.items():
print(f"'{key}': {value['messages'][-1]}")
print("~~~~~~~~")
# Execute the integration
asyncio.run(run_with_zep())
Initialization:
- Zep initialization: The AsyncZep client is initialized using an API key, establishing a connection to Zep's memory services.
- State definition: The State class defines the structure of the data maintained by LangGraph, including messages, user identifiers, and session IDs.
Tool definition:
- search_facts function: This asynchronous function interacts with Zep to search for relevant facts based on user queries. It leverages Zep's search_sessions method to retrieve pertinent information from stored sessions.
Chatbot function:
- chatbot function: This function retrieves relevant facts from Zep using the session ID and constructs a response that includes this historical context. If no facts are available, it indicates so.
Graph construction:
- StateGraph initialization: A StateGraph is created with the defined State.
- Node addition: The chatbot and search facts tools are added as nodes to the graph.
- Edge definition: Edges are established to define the workflow. The chatbot node directs the flow to the search facts node if the user input contains the word "search"; otherwise, the workflow ends.
Running the graph:
- run_with_zep function: This asynchronous function sets up a unique user and session, adds them to Zep, and defines the initial user input. It then streams the outputs from the graph, printing responses as generated.
For more detailed insights into how Zep leverages AI knowledge graphs for memory management, refer to Zep's blog on AI Knowledge Graph Memory. This provides an in-depth look at the benefits of using knowledge graphs to enhance AI memory systems.
How memory will evolve in AI Applications
As AI technologies advance, the role of memory systems becomes increasingly vital in creating context-aware and personalized experiences. Here are some key points:
Context and personalization
Long-term memory is essential for providing personalized and contextually relevant interactions. AI agents that can remember past interactions and user preferences can deliver more tailored responses, improving user satisfaction and engagement. This capability is particularly important for applications like virtual assistants, customer support, and personalized learning tools, where understanding the user's history can significantly enhance the interaction quality.
Role of Zep
Zep is significantly improving memory systems within LangGraph. Its focus on privacy ensures that user data is handled securely, addressing one of the critical concerns in AI development.
Looking ahead, developers will need to implement strategies for pruning and selecting relevant history to prevent the accumulation of excessive data, which can hinder performance.
Techniques like summarization and selective retention will be essential to balance memory depth with efficiency. Additionally, robust privacy measures will remain a priority, ensuring that user data is protected while enabling meaningful interactions.
Limitations of LangGraph
While LangGraph offers a robust framework for building complex AI workflows, it is not without its challenges. Understanding these limitations is crucial for developers to make informed decisions about its adoption.
Complexity of setup
One of the primary drawbacks of LangGraph is its complexity during the initial setup. Unlike LangChain, which is relatively straightforward to configure for simple task chains, LangGraph requires a deeper understanding of graph-based architectures and state management.
Developers need to define state structures, nodes, and edges, which can be time-consuming and may present a steep learning curve, especially for those new to graph-oriented frameworks.
Agent looping
A significant concern with LangGraph is the potential for agents to unintentionally create loops. If an agent sends outputs back to itself without proper control mechanisms, it can result in infinite loops.
This not only increases the runtime but also leads to higher token consumption, which can be costly and inefficient. Such scenarios require developers to implement safeguards to prevent agents from getting stuck in repetitive cycles.
Performance impact
Unmanaged cycles and complex workflows can degrade the overall performance of applications built with LangGraph.
Each loop or conditional branch consumes additional resources, potentially slowing down the application and increasing operational costs. Developers must design workflows carefully to avoid unnecessary loops and optimize resource usage, ensuring that the application remains both efficient and cost-effective. In a recent update of the LangGraph Python library, performance enhancements and CI benchmarks were introduced to optimize workflow efficiency and address some of the previously noted limitations, such as resource usage and scalability challenges.
{{banner-small-1="/banners"}}
Last thoughts on LangChain vs LangGraph
LangChain and LangGraph each bring unique strengths, making them suitable for different types of AI workflows.
LangChain is ideal for simpler, linear task chains, offering flexibility and modularity. In contrast, LangGraph shines in managing more complex workflows that require advanced orchestration and state management. The ability to handle persistent states and incorporate human-in-the-loop workflows further enhances its capability to build sophisticated AI agents.
Advanced memory management in LangGraph, particularly through integrations with tools like Zep, adds significant value. These memory systems enable AI agents to maintain context and personalize interactions over extended periods, which is crucial for delivering consistent and user-centric experiences.
By understanding the differences between LangChain and LangGraph, developers can choose the framework that best aligns with their project requirements, ensuring the creation of effective, scalable, and intelligent AI solutions.